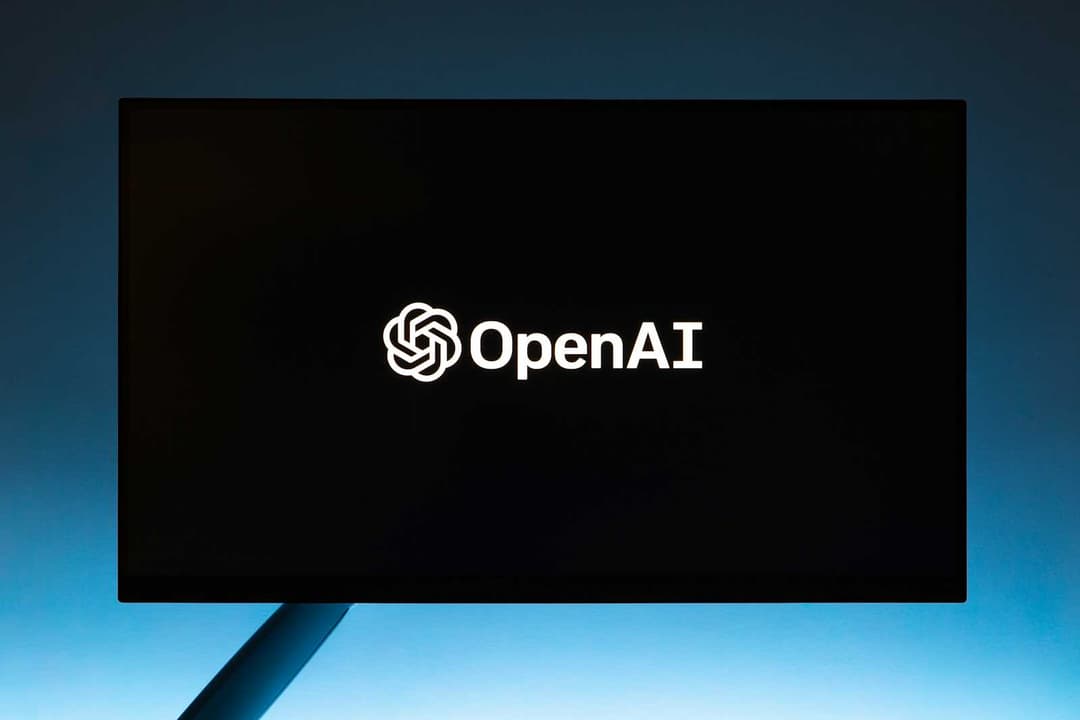
Unlocking the Future: Programming with AI
Artificial Intelligence (AI) is revolutionizing the way we approach software development. It allows developers to build smarter applications that can learn, adapt, and make decisions without explicit instructions. This blog will guide you through the basics of AI in programming and provide insight into how to integrate AI into your projects. Why AI in Programming? AI offers unprecedented advantages in application development: Automation: AI can handle repetitive tasks such as data sorting, image classification, and even code generation. Intelligence: AI enables applications to process massive datasets, understand patterns, and provide intelligent responses. Personalization: AI can tailor user experiences based on behavior analysis, providing a custom touch to apps. Scalability: With AI-driven automation, systems can scale to manage larger data sets and user bases more efficiently. Getting Started with AI in Programming 1. Choose Your AI Tools AI programming requires libraries and frameworks to handle complex algorithms and data processing. Here are some popular AI tools: TensorFlow: A comprehensive open-source platform by Google for machine learning. PyTorch: A flexible, deep learning library popular for research and prototyping. Keras: A high-level neural networks API that runs on top of TensorFlow or Theano. OpenAI GPT: A powerful natural language processing (NLP) model, ideal for tasks like chatbots, text generation, and summarization. 2. Understanding AI Concepts Before diving into code, it's important to grasp core AI concepts: Machine Learning (ML): A branch of AI that involves teaching systems to learn from data. Neural Networks: The backbone of deep learning, simulating how the human brain processes information. Natural Language Processing (NLP): AI's ability to interpret and respond to human language, enabling tools like virtual assistants. Computer Vision: AI's capability to analyze and interpret visual information, such as images or videos. Building an AI-Powered Application Let’s create a simple example: a movie recommendation system using AI. Step 1: Setting Up Your Environment You'll need Python installed on your system, along with the following libraries: bashCopy codepip install numpy pandas scikit-learn tensorflow Numpy and Pandas will help manage and manipulate data. Scikit-learn will handle the machine learning algorithms. TensorFlow will manage neural networks and deep learning tasks. Step 2: Preparing Data We'll use a dataset of movies and user ratings. Here’s an example structure for the data: UserIDMovieIDRating 11004 21015 31023 We’ll train a machine learning model using this data to predict future movie ratings for different users. pythonCopy codeimport pandas as pd # Load dataset data = pd.read_csv("movie_ratings.csv") # Prepare data X = data[['UserID', 'MovieID']] y = data['Rating'] Step 3: Building the AI Model We'll use a simple neural network to predict movie ratings based on user and movie IDs: pythonCopy codefrom tensorflow.keras.models import Sequential from tensorflow.keras.layers import Dense, Embedding, Flatten # Build the neural network model = Sequential() model.add(Embedding(input_dim=10000, output_dim=64, input_length=2)) model.add(Flatten()) model.add(Dense(1, activation='linear')) model.compile(optimizer='adam', loss='mean_squared_error') # Train the model model.fit(X, y, epochs=10) Step 4: Making Predictions Once trained, we can use the model to predict movie ratings: pythonCopy code# Example: Predict rating for User 1 and Movie 100 prediction = model.predict([[1, 100]]) print(f"Predicted Rating: {prediction[0][0]}") This is a simple example of how AI can be applied to a recommendation system, but the possibilities are endless! AI Use Cases in Programming Natural Language Processing: From chatbots to AI content generators, NLP is at the forefront of many modern applications. Computer Vision: Face recognition, object detection, and even augmented reality (AR) apps use AI to process images and videos. Speech Recognition: Applications like virtual assistants and automated transcription tools rely heavily on AI for converting speech to text. Future of AI in Programming AI's future in software development is limitless. AI programming is not just about making existing processes smarter—it's about creating entirely new ways of interacting with technology. Whether it's autonomous driving, AI-generated art, or self-learning algorithms, AI is paving the way for a future where machines can understand, learn, and create alongside humans. Conclusion Integrating AI into your programming projects can open up a world of possibilities. From automation to personalization, AI-driven applications can enhance user experiences and optimize processes in ways traditional programming cannot. With the right tools and understanding of AI concepts, you're set to embark on your journey into the future of development. Stay tuned for more in-depth AI tutorials and guides on how to integrate advanced AI techniques into your code!
read more ...